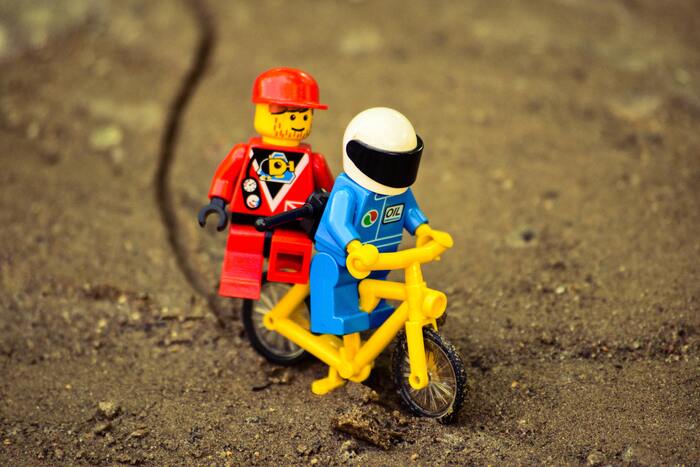
Run c# inside powershell
Powershell is quite powerful already for most purposes. Yet there are times when you need to use features that are not available in powershell directly. An example would be interacting with the OS via DllImports (like Windows API).
Luckily, powershell has the ability to compile c# inline using the Add-Type command (https://docs.microsoft.com/en-us/powershell/module/microsoft.powershell.utility/add-type?view=powershell-7.2).
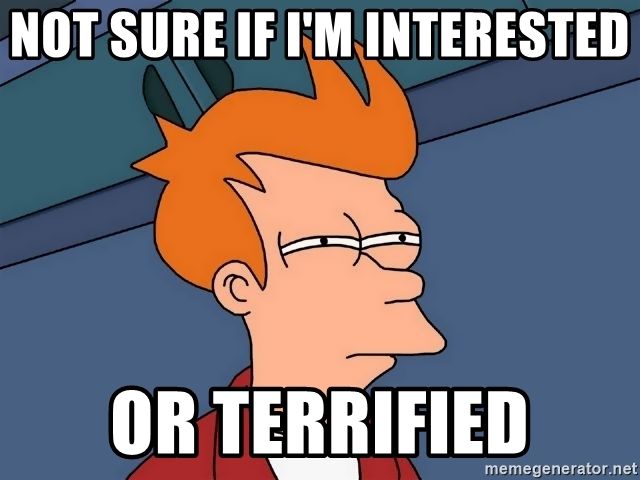
As an example we're just using a static class in c# and importing the type.
$source = @"
using System;
using System.Runtime.InteropServices;
namespace Demo
{
public static class Calc
{
public static int Add(int a, int b) { return a + b; }
}
}
"@
# import as types
Add-Type -TypeDefinition $source -Language CSharp
Write-output "Adding two numbers. 37+5=$([Demo.Calc]::Add(37,5))"
This is quite easy. The output is as follows:
.\calc.ps1
Adding two numbers. 37+5=42
Create class instances
Of course you can also create an instance of a class as usual. Here's a short example that covers this:
$source = @"
using System;
using System.Runtime.InteropServices;
namespace Demo
{
public class Car
{
public bool IsDriving { get; set; }
public void Start() { IsDriving = true; }
public void Stop() { IsDriving = false; }
}
}
"@
# import as types
Add-Type -TypeDefinition $source -Language CSharp
Write-output "Creating a new car"
$car = New-Object Demo.Car
Write-output "Starting car"
$car.Start();
Write-output "Car is driving: $($car.IsDriving)"
Write-output "Stopping car"
$car.Stop();
Write-output "Car is driving: $($car.IsDriving)"
The output shows that we can handle the state:
.\car.ps1
Creating a new car
Starting car
Car is driving: True
Stopping car
Car is driving: False
Is there even more?
Sure there is ton's of additional stuff you can use, like loading types from assemblies, referencing assemblies and so on. So, if you want to reuse dotnet assemblies or add complex code logic you've already implemented, take a look add the docs: https://docs.microsoft.com/en-us/powershell/module/microsoft.powershell.utility/add-type?view=powershell-7.2